Basic Full Stack Python Developer Interview Questions for Beginners
Answer: The `strip()` method is used to remove leading and trailing whitespace from a string (or other specified characters), while the `replace()` method is used to replace occurrences of a substring within the string with another substring. For example, `” Hello “.strip()` results in `”Hello”`, and `”Hello”.replace(‘e’, ‘a’)` results in `”Hallo”`.
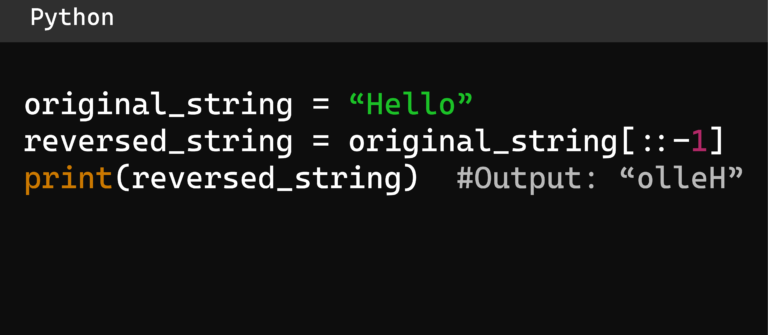
3. Explain the difference between the `==` operator and the `is` operator.
Answer: The `==` operator checks if the values of two variables are equal, whereas the `is` operator checks whether both variables point to the same object in memory. For example, `list1 = []` and `list2 = []` are equal (`list1 == list2` is `True`), but they are not the same object (`list1 is list2` is `False`).

5. How do you check the presence of a character in a string?
Intermediate Full Stack Python Developer Interview Questions
6. What is the output of the expression `3.5 + 2` and why?
Answer: The output of the expression `3.5 + 2` is `5.5`. This is because Python performs automatic type conversion, adding the integer `2` to the floating-point number `3.5`, resulting in a floating-point sum
Get Skilled
7. How can you format the string “Hi {name}. You are {age} years old.” using Python’s f-string?
Answer: You can format this string using an f-string as follows:
“`python
name = “John”
age = 30
print(f”Hi {name}. You are {age} years old.”) “`
This will output: `”Hi John. You are 30 years old.”`
8. What is the result of `len(“Hello World“)` and what does `len()` compute?
Answer: The result of `len(“Hello World”)` is `11`. The `len()` function computes the number of items in an object, which in the case of a string is the number of characters, including spaces.

9. Explain the use of the modulus operator in Python with an example.
Answer: The modulus operator `%` is used to find the remainder of a division. For example, `7 % 3` would return `1`, because 7 divided by 3 leaves a remainder of 1.
10. Describe how you would use a loop to iterate over a dictionary and print each key-value pair.
Answer: You can iterate over a dictionary using a for loop to print each key-value pair as follows:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
for key, value in my_dict.items():
print(f”Key: {key}, Value: {value}”) “`
This loop uses the `.items()` method of the dictionary to return pairs of keys and values, which are then printed in each iteration.
Advanced Full Stack Python Developer Interview Questions
11. What is the purpose of a while loop in Python?
Answer: A while loop in Python allows for repeated execution of a block of code as long as a specified condition remains true. It is used when the number of iterations is not predetermined before the start of the loop.
12. How does a for loop differ from a while loop?
Answer: A for loop in Python iterates over items of a sequence (like a list, tuple, or string), executing a block of code for each item. A while loop, on the other hand, continues to execute as long as a condition evaluates to True, making it suitable for scenarios where the number of iterations isn’t known in advance.
13. Explain the use of the range function in a for loop with an example.
Answer: The range function generates a sequence of numbers. It is commonly used in for loops to execute the loop body a certain number of times. For example, `for i in range(5)` will execute the loop body 5 times, iterating over i values 0 to 4.
14. How can you concatenate two lists in Python?
Answer: Two lists can be concatenated using the `+` operator. For example, `list_a = [1, 2]` and `list_b = [“a”, “b“]` can be concatenated as `list_c = list_a + list_b` resulting in `list_c` being `[1, 2, ‘a’, ‘b‘]`.
15. Describe list slicing with an example.
Answer: List slicing in Python allows you to obtain a part of the list. For instance, `list_a = [1, 2, 3, 4, 5]`, slicing `list_a[1:4]` would produce `[2, 3, 4]`. Slicing syntax is `list[start:stop:step]`, where `start` is inclusive and `stop` is exclusive.